Beautiful Soup¶
First steps¶
Beautiful Soup is a Python library for retrieving data from HTML (and other XML) files. We will explore its basic functionality using the UB Math Department web page with graduate student directory as an example:
https://www.buffalo.edu/cas/math/people/grad-directory.html
[1]:
# display the web page in the notebook
import IPython
url = "https://www.buffalo.edu/cas/math/people/grad-directory.html"
IPython.display.IFrame(url, width=720, height=600)
[1]:
Note: This is a live web page, and it is regularly updated. If you use the code below, your output may differ to some extent from the one shown here.
To start, we will use requests
to get the HTML code of the page:
[3]:
import requests
grad_page = requests.get("http://www.buffalo.edu/cas/math/people/grad-directory.html").text
print(grad_page[:1000])
<!DOCTYPE HTML><html lang="en" class="ubcms-65"><!-- cmspub03 0310-231422 --><head><link rel="preconnect" hre
f="https://www.googletagmanager.com/" crossorigin/><link rel="dns-prefetch" href="https://www.googletagmanage
r.com/"/><link rel="dns-prefetch" href="https://connect.facebook.net/"/><link rel="dns-prefetch" href="https:
//www.google-analytics.com/"/><meta http-equiv="X-UA-Compatible" content="IE=edge"/><meta http-equiv="content
-type" content="text/html; charset=UTF-8"/><meta id="meta-viewport" name="viewport" content="width=device-wid
th,initial-scale=1"/><script>if (screen.width > 720 && screen.width < 960) document.getElementById('meta-view
port').setAttribute('content','width=960');</script><script>(function(w,d,s,l,i){w[l]=w[l]||[];w[l].push({'gt
m.start':new Date().getTime(),event:'gtm.js'});var f=d.getElementsByTagName(s)[0],j=d.createElement(s),dl=l!=
'dataLayer'?'&l='+l:'';j.async=true;j.src='https://www.googletagmanager.com/gtm.js?id='+i+dl;f.parentNode.ins
ertBefore(j,f);})(w
Next, we import BeautifulSoup
and pass to it the HTML code of the web page to produce a BeautifulSoup
object:
[5]:
from bs4 import BeautifulSoup
soup = BeautifulSoup(grad_page)
One useful feature of this new object is that it can produce a nicer printout of the HTML code:
[6]:
print(soup.prettify())
<!DOCTYPE HTML>
<html class="ubcms-65" lang="en">
<!-- cmspub03 0310-231422 -->
<head>
<link crossorigin="" href="https://www.googletagmanager.com/" rel="preconnect"/>
<link href="https://www.googletagmanager.com/" rel="dns-prefetch"/>
<link href="https://connect.facebook.net/" rel="dns-prefetch"/>
<link href="https://www.google-analytics.com/" rel="dns-prefetch"/>
<meta content="IE=edge" http-equiv="X-UA-Compatible"/>
<meta content="text/html; charset=utf-8" http-equiv="content-type"/>
<meta content="width=device-width,initial-scale=1" id="meta-viewport" name="viewport"/>
<script>
if (screen.width > 720 && screen.width < 960) document.getElementById('meta-viewport').setAttribute('content','width=960');
</script>
<script>
(function(w,d,s,l,i){w[l]=w[l]||[];w[l].push({'gtm.start':new Date().getTime(),event:'gtm.js'});var f=d.getElementsByTagName(s)[0],j=d.createElement(s),dl=l!='dataLayer'?'&l='+l:'';j.async=true;j.src='https://www.googletagmanager.com/gtm.js?id='+i+dl;f.parentNode.insertBefore(j,f);})(window,document,'script','dataLayer','GTM-T5KRRKT');
</script>
<title>
Graduate Student Directory - Department of Mathematics - University at Buffalo
</title>
<link href="https://www.buffalo.edu/cas/math/people/grad-directory.html" rel="canonical"/>
<meta content="2022-03-01" name="date"/>
<meta content="Graduate Student Directory" property="og:title"/>
<meta content=" A | B | C | D | E | F | G | H | I | J | K | L | M | N | O | P | Q | R | S | T | U | V | W | X | Y | Z " property="og:description"/>
<meta content="https://www.buffalo.edu/etc/designs/ubcms/clientlibs-main/images/ub-social.png.img.512.auto.png/1615975613913.png" property="og:image"/>
<meta content="University at Buffalo" property="og:image:alt"/>
<meta content="summary_large_image" name="twitter:card"/>
<meta content="https://www.buffalo.edu/cas/math/people/grad-directory/_jcr_content/par/image.img.512.auto.jpg/1629919606956.jpg" property="thumbnail"/>
<meta content="1. " property="thumbnail:alt"/>
<link href="/v-5150cc622c68590719981526ed5c6b25/etc/designs/ubcms/clientlibs-privateauthor.min.5150cc622c68590719981526ed5c6b25.css" rel="stylesheet" type="text/css"/>
<link href="/v-3d5e50c59343478ac1f797325d06d08c/etc/designs/ubcms/clientlibs.min.3d5e50c59343478ac1f797325d06d08c.css" rel="stylesheet" type="text/css"/>
<link href="/v-9cc4b89851550cf5e105d25db85ba3e9/etc/designs/cas/math/css/main.css" rel="stylesheet" type="text/css"/>
<script nomodule="" src="/v-a83c7a045b4a3c7a9600758298bc4ad8/etc/designs/ubcms/clientlibs-polyfills.min.a83c7a045b4a3c7a9600758298bc4ad8.js">
</script>
<script src="/etc.clientlibs/clientlibs/granite/jquery.min.cee8557e8779d371fe722bbcdd3b3eb7.js">
</script>
<script src="/v-7cc18f24035dfccf28f4dfe0af578c03/etc/designs/ubcms/clientlibs.min.7cc18f24035dfccf28f4dfe0af578c03.js">
</script>
<style>
body.page #page, body.page .page-inner {background-color:#FFFFFF}
</style>
<script>
(function(i,s,o,g,r,a,m){i['GoogleAnalyticsObject']=r;i[r]=i[r]||function(){(i[r].q=i[r].q||[]).push(arguments)},i[r].l=1*new Date();a=s.createElement(o),m=s.getElementsByTagName(o)[0];a.async=1;a.src=g;m.parentNode.insertBefore(a,m)})(window,document,'script','//www.google-analytics.com/analytics.js','ga');ga('create', 'UA-67291618-1', 'auto');ga('send', 'pageview');
</script>
<style>
img.lazyload,img.lazyloading{position:relative;background:#EEE}
img.lazyload:before,img.lazyloading:before{content:"";background:#EEE;position:absolute;top:0;left:0;bottom:0;right:0}
</style>
</head>
<body class="contentpage page">
<noscript>
<iframe height="0" src="https://www.googletagmanager.com/ns.html?id=GTM-T5KRRKT" style="display:none;visibility:hidden" width="0">
</iframe>
</noscript>
<nav aria-label="skip to content">
<a href="#skip-to-content" id="skip-to-content-link">
Skip to Content
</a>
</nav>
<div>
</div>
<div id="page">
<div class="page-inner">
<div class="page-inner-1">
<div class="page-inner-2">
<div class="page-inner-2a">
</div>
<div class="page-inner-3">
<header>
<div class="innerheader inheritedreference reference parbase">
<div class="headerconfigpage contentpage page basicpage">
<div class="par parsys">
<div class="alertbanner reference parbase section">
<div contenttreeid="alertbanner" contenttreestatus="Not published" style="display:none;">
</div>
<script>
jQuery('.cap-message').detach().insertBefore('#page').wrap('<section aria-label="UB Alert">');
</script>
</div>
<div class="header section">
<style>
.innerheader { padding-top: 158px }
.header { height: 158px }
.header .main { height: 134px }
</style>
<div>
<div class="top theme-blue" data-set="header-links">
<ul class="school-links">
<li>
<a href="http://arts-sciences.buffalo.edu/">
College of Arts and Sciences
</a>
</li>
</ul>
<ul class="pervasive-links">
<li>
<a href="//www.buffalo.edu">
UB Home
</a>
</li>
<li>
<a href="//www.buffalo.edu/maps">
Maps
</a>
</li>
<li>
<a href="//www.buffalo.edu/directory/">
UB Directory
</a>
</li>
</ul>
</div>
<div class="main theme-blue brand-extension lines-1">
<div class="lockup">
<a href="//www.buffalo.edu">
<i class="icon icon-ub-logo">
</i>
<span class="ada-hidden">
University at Buffalo
</span>
<span class="logo">
<img alt="" class="black" height="20" src="/v-be9166b6b4a1ea7e5771e2eba1d410cf/etc.clientlibs/wci/components/block/header/clientlibs/resources/ub-logo-black.png" width="181"/>
</span>
</a>
<div class="mobile-links" data-set="header-links">
</div>
<a class="title" href="/cas/math.html">
Department of Mathematics
</a>
</div>
<div class="social" data-set="headersocial">
</div>
<div class="tasknav" data-set="tasknav">
<div class="buttoncomponent sidebyside orange">
<a href="http://www.buffalo.edu/ub_admissions/apply-now.html" target="_blank">
Apply to UB
</a>
</div>
<div class="buttoncomponent sidebyside blue">
<a href="http://www.buffalo.edu/cas/math/about-us/contact-us.html">
Contact Us
</a>
</div>
<div class="buttoncomponent sidebyside green">
<a href="https://ubfoundation.buffalo.edu/giving/?gift_allocation=01-3-0-01240" target="_blank">
Support UB Math
</a>
</div>
</div>
</div>
</div>
</div>
<div class="reference parbase section">
<div class="unstructuredpage page basicpage">
<div class="par parsys">
<div class="list parbase section">
<div data-columnize-row="1" id="ubcms-gen-33853657">
<ul class="list-style-detail" data-columnize="1">
<li>
<div class="long-term-alert-banner">
<div class="text parbase section">
<p>
<b>
COVID-19 UPDATES • 3/4/2022
</b>
</p>
<ul>
<li>
<a href="/coronavirus/latest-update.html">
UB lifts vaccination policy for campus events
</a>
</li>
</ul>
</div>
</div>
</li>
</ul>
</div>
<script>
UBCMS.list.listlimit('ubcms\u002Dgen\u002D33853657', '100',
'100');
</script>
</div>
<script>
UBCMS.longTermAlert.init()
</script>
</div>
</div>
<div contenttreeid="reference" contenttreestatus="Not published" style="display:none;">
</div>
</div>
<div class="mobilemenu section">
<!--noindex-->
<nav aria-label="mobile navigation menu">
<ul>
<li class="mobileheader-button-menu" style="display: none">
<a href="#">
Menu
</a>
</li>
<li class="mobileheader-button-search" style="display: none">
<a href="#">
Search
</a>
</li>
</ul>
</nav>
<!--endnoindex-->
<div class="menu" id="mobile-search">
<div class="menu-inner" data-set="mobile-search" id="mobile-search-inner">
</div>
</div>
<div class="menu" id="mobile-menu">
<div class="menu-inner">
<div id="mobile-menu-inner">
<div class="loading">
<!--noindex-->
Loading menu...
<!--endnoindex-->
</div>
</div>
<div class="tasknav" data-set="tasknav">
</div>
<div class="headersocial" data-set="headersocial">
</div>
</div>
</div>
<script>
jQuery('.mobileheader-button-menu').removeAttr('style');
jQuery(document).ready(function() {
if ($('.topnav .search .search-content').length > 0) {
$('.mobileheader-button-search').removeAttr('style');
}
});
UBCMS.rwd.mobileMenu.load('\/content\/cas\/math\/jcr:content.nav.html', 'https:\/\/www.buffalo.edu\/cas\/math\/people\/grad-directory.html');
</script>
</div>
<div class="topnav section">
<nav aria-label="site navigation" class="topnav-inner">
<div class="main">
<ul class="menu">
<li class="first theme-secondary theme-standard-gray">
<a aria-haspopup="true" href="/cas/math/about-us.html" id="ubcms-gen-33853660">
<span class="container">
About
</span>
</a>
<div aria-labelledby="ubcms-gen-33853660" class="topnav-submenu-container">
<ul class="submenu clearfix">
<li class="first">
<a aria-label="About:Why Choose Us?" href="/cas/math/about-us/why-choose.html">
Why Choose Us?
</a>
</li>
<li>
<a aria-label="About:Our Mission" href="/cas/math/about-us/our-mission.html">
Our Mission
</a>
</li>
<li>
<a aria-label="About:Our Alumni, Students, and Faculty" href="/cas/math/about-us/our-alumni.html">
Our Alumni, Students, and Faculty
</a>
<div class="topnav-submenu-children-container list">
<ul class="submenu-children clearfix link-list">
<li class="first">
<a aria-label="Our Alumni, Students, and Faculty:Our Alumni" href="/cas/math/about-us/our-alumni/our-alumni.html">
Our Alumni
</a>
</li>
<li>
<a aria-label="Our Alumni, Students, and Faculty:Our Students" href="/cas/math/about-us/our-alumni/our-students.html">
Our Students
</a>
</li>
<li class="last">
<a aria-label="Our Alumni, Students, and Faculty:Our Faculty" href="/cas/math/about-us/our-alumni/our-faculty.html">
Our Faculty
</a>
</li>
</ul>
</div>
</li>
<li>
<a aria-label="About:Memberships" href="/cas/math/about-us/memberships.html">
Memberships
</a>
<div class="topnav-submenu-children-container list">
<ul class="submenu-children clearfix link-list">
<li class="first">
<a aria-label="Memberships:American Mathematical Society" href="/cas/math/about-us/memberships/AMS.html">
American Mathematical Society
</a>
</li>
<li>
<a aria-label="Memberships:Association for Women in Mathematics" href="/cas/math/about-us/memberships/AWM.html">
Association for Women in Mathematics
</a>
</li>
<li class="last">
<a aria-label="Memberships:Mathematical Sciences Research Institute" href="/cas/math/about-us/memberships/MSRI.html">
Mathematical Sciences Research Institute
</a>
</li>
</ul>
</div>
</li>
<li>
<a aria-label="About:Mathematicians of the African Diaspora" href="/cas/math/about-us/mathematicians-of-the-african-diaspora.html">
Mathematicians of the African Diaspora
</a>
</li>
<li>
<a aria-label="About:About the University" href="/cas/math/about-us/about-the-university.html">
About the University
</a>
</li>
<li>
<a aria-label="About:About Buffalo-Niagara" href="/cas/math/about-us/the-buffalo-niagara-region.html">
About Buffalo-Niagara
</a>
</li>
<li>
<a aria-label="About:Visiting UB" href="/cas/math/news-events/visiting.html">
Visiting UB
</a>
</li>
<li class="last">
<a aria-label="About:Contact Us" href="/cas/math/about-us/contact-us.html">
Contact Us
</a>
</li>
</ul>
<div class="relatedLinks relatedlinksreference reference parbase">
</div>
</div>
</li>
<li class="theme-secondary theme-standard-gray active-trail">
<a aria-haspopup="true" href="/cas/math/people.html" id="ubcms-gen-33853662">
<span class="container">
People
</span>
</a>
<div aria-labelledby="ubcms-gen-33853662" class="topnav-submenu-container">
<ul class="submenu clearfix">
<li class="first">
<a aria-label="People:Faculty" href="/cas/math/people/faculty.html">
Faculty
</a>
</li>
<li>
<a aria-label="People:Staff" href="/cas/math/people/staff_directory.html">
Staff
</a>
</li>
<li>
<a aria-label="People:Emeriti Faculty" href="/cas/math/people/emeriti.html">
Emeriti Faculty
</a>
</li>
<li>
<a aria-label="People:Instructors" href="/cas/math/people/instructors.html">
Instructors
</a>
</li>
<li>
<a aria-label="People:Visiting Scholars" href="/cas/math/people/visiting-scholars.html">
Visiting Scholars
</a>
</li>
<li class="active-trail">
<a aria-label="People:Graduate Student Directory" class="active" href="/cas/math/people/grad-directory.html">
Graduate Student Directory
</a>
</li>
<li class="last">
<a aria-label="People:Alumni" href="/cas/math/people/alumni-friends.html">
Alumni
</a>
<div class="topnav-submenu-children-container list">
<ul class="submenu-children clearfix link-list">
<li class="first">
<a aria-label="Alumni:Class of 2021" href="/cas/math/people/alumni-friends/class-of-2021.html">
Class of 2021
</a>
</li>
<li>
<a aria-label="Alumni:Class of 2020" href="/cas/math/people/alumni-friends/class-of-2020.html">
Class of 2020
</a>
</li>
<li class="last">
<a aria-label="Alumni:PhD Recipients, 2010 to Present" href="/cas/math/grad/PhD-recipients.html">
PhD Recipients, 2010 to Present
</a>
</li>
</ul>
</div>
</li>
</ul>
<div class="relatedLinks relatedlinksreference reference parbase">
</div>
</div>
</li>
<li class="theme-secondary theme-standard-gray">
<a aria-haspopup="true" href="/cas/math/research.html" id="ubcms-gen-33853664">
<span class="container">
Research
</span>
</a>
<div aria-labelledby="ubcms-gen-33853664" class="topnav-submenu-container">
<ul class="submenu clearfix">
<li class="first">
<a aria-label="Research:Algebra" href="/cas/math/research/algebra.html">
Algebra
</a>
</li>
<li>
<a aria-label="Research:Analysis" href="/cas/math/research/analysis.html">
Analysis
</a>
</li>
<li>
<a aria-label="Research:Applied Mathematics" href="/cas/math/research/applied-mathematics.html">
Applied Mathematics
</a>
</li>
<li>
<a aria-label="Research:Geometry and Topology " href="/cas/math/research/geometry-topology.html">
Geometry and Topology
</a>
</li>
<li class="last">
<a aria-label="Research:Undergraduate Research" href="/cas/math/ug/undergraduate-research.html">
Undergraduate Research
</a>
</li>
</ul>
<div class="relatedLinks relatedlinksreference reference parbase">
</div>
</div>
</li>
<li class="theme-secondary theme-standard-gray">
<a aria-haspopup="true" href="/cas/math/ug.html" id="ubcms-gen-33853666">
<span class="container">
Undergraduate
</span>
</a>
<div aria-labelledby="ubcms-gen-33853666" class="topnav-submenu-container">
<ul class="submenu clearfix">
<li class="first">
<a aria-label="Undergraduate:Undergraduate Programs" href="/cas/math/ug/undergraduate-programs.html">
Undergraduate Programs
</a>
</li>
<li>
<a aria-label="Undergraduate:Undergraduate Research" href="/cas/math/ug/undergraduate-research.html">
Undergraduate Research
</a>
<div class="topnav-submenu-children-container list">
<ul class="submenu-children clearfix link-list">
<li class="first">
<a aria-label="Undergraduate Research:Special Research Projects" href="/cas/math/ug/undergraduate-research/special-projects.html">
Special Research Projects
</a>
</li>
<li class="last">
<a aria-label="Undergraduate Research:Summer Math Scholarship" href="/cas/math/ug/undergraduate-research/summer.html">
Summer Math Scholarship
</a>
</li>
</ul>
</div>
</li>
<li>
<a aria-label="Undergraduate:Honors, Awards, and Scholarships" href="/cas/math/ug/honors--awards--and-scholarships.html">
Honors, Awards, and Scholarships
</a>
</li>
<li>
<a aria-label="Undergraduate:Undergraduate Courses" href="/cas/math/ug/ug-courses.html">
Undergraduate Courses
</a>
<div class="topnav-submenu-children-container list">
<ul class="submenu-children clearfix link-list">
<li class="first">
<a aria-label="Undergraduate Courses:Sample Syllabi" href="/cas/math/ug/ug-courses/syllabi.html">
Sample Syllabi
</a>
</li>
<li>
<a aria-label="Undergraduate Courses:MTH 121 and 122 Textbook" href="/cas/math/ug/ug-courses/mth121-122-textbook.html">
MTH 121 and 122 Textbook
</a>
</li>
<li class="last">
<a aria-label="Undergraduate Courses:MTH 306 Textbook" href="/cas/math/ug/ug-courses/mth-306-textbook.html">
MTH 306 Textbook
</a>
</li>
</ul>
</div>
</li>
<li>
<a aria-label="Undergraduate:Directed Reading Program" href="https://sites.google.com/view/ubmathdrp/home">
Directed Reading Program
</a>
</li>
<li>
<a aria-label="Undergraduate:Mathematics Resources" href="/cas/math/ug/resources.html">
Mathematics Resources
</a>
<div class="topnav-submenu-children-container list">
<ul class="submenu-children clearfix link-list">
<li class="first">
<a aria-label="Mathematics Resources:Mathematics Placement Exam" href="/cas/math/ug/resources/mathplacement.html">
Mathematics Placement Exam
</a>
</li>
<li>
<a aria-label="Mathematics Resources:Mathematics Placement Exam Frequently Asked Questions" href="/cas/math/ug/resources/MPEFAQ.html">
Mathematics Placement Exam Frequently Asked Questions
</a>
</li>
<li class="last">
<a aria-label="Mathematics Resources:Force Registration" href="/cas/math/ug/resources/force-registration.html">
Force Registration
</a>
</li>
</ul>
</div>
</li>
<li>
<a aria-label="Undergraduate:Mathematics Help Center" href="/cas/math/ug/help-center.html">
Mathematics Help Center
</a>
<div class="topnav-submenu-children-container list">
<ul class="submenu-children clearfix link-list">
<li class="first last">
<a aria-label="Mathematics Help Center:Online Help Sessions" href="/content/cas/math/ug/help-center/zoom-pw.html">
Online Help Sessions
</a>
</li>
</ul>
</div>
</li>
<li class="last">
<a aria-label="Undergraduate:Association for Women in Mathematics" href="/cas/math/about-us/memberships/AWM.html">
Association for Women in Mathematics
</a>
</li>
</ul>
<div class="relatedLinks relatedlinksreference reference parbase">
</div>
</div>
</li>
<li class="theme-secondary theme-standard-gray">
<a aria-haspopup="true" href="/cas/math/grad.html" id="ubcms-gen-33853668">
<span class="container">
Graduate
</span>
</a>
<div aria-labelledby="ubcms-gen-33853668" class="topnav-submenu-container">
<ul class="submenu clearfix">
<li class="first">
<a aria-label="Graduate:Master's Program" href="/cas/math/grad/master-program.html">
Master's Program
</a>
</li>
<li>
<a aria-label="Graduate:Doctoral Program (PhD)" href="/cas/math/grad/doctoral-program.html">
Doctoral Program (PhD)
</a>
</li>
<li>
<a aria-label="Graduate:Request Information" href="/cas/math/grad/request-information.html">
Request Information
</a>
</li>
<li>
<a aria-label="Graduate:Admissions" href="/cas/math/grad/grad-admissions.html">
Admissions
</a>
</li>
<li>
<a aria-label="Graduate:Courses" href="/cas/math/grad/grad-courses.html">
Courses
</a>
</li>
<li>
<a aria-label="Graduate:Directed Reading Program" href="https://sites.google.com/view/ubmathdrp/home">
Directed Reading Program
</a>
</li>
<li>
<a aria-label="Graduate:Graduate Research" href="/cas/math/grad/grad-research.html">
Graduate Research
</a>
</li>
<li>
<a aria-label="Graduate:Fellowships, Scholarships, Awards" href="/cas/math/grad/fellowships-awards.html">
Fellowships, Scholarships, Awards
</a>
</li>
<li>
<a aria-label="Graduate:PhD Recipients, 2010 to present" href="/cas/math/grad/PhD-recipients.html">
PhD Recipients, 2010 to present
</a>
</li>
<li>
<a aria-label="Graduate:Association for Women in Mathematics" href="/cas/math/about-us/memberships/AWM.html">
Association for Women in Mathematics
</a>
</li>
<li>
<a aria-label="Graduate:Graduate Student Lecture Series" href="/cas/math/grad/gsls.html">
Graduate Student Lecture Series
</a>
</li>
<li class="last active-trail">
<a aria-label="Graduate:Graduate Student Directory" href="/cas/math/people/grad-directory.html">
Graduate Student Directory
</a>
</li>
</ul>
<div class="relatedLinks relatedlinksreference reference parbase">
</div>
</div>
</li>
<li class="theme-secondary theme-standard-gray">
<a aria-haspopup="true" href="/cas/math/courses.html" id="ubcms-gen-33853670">
<span class="container">
Courses
</span>
</a>
</li>
<li class="last theme-secondary theme-standard-gray">
<a aria-haspopup="true" href="/cas/math/news-events/news.html" id="ubcms-gen-33853671">
<span class="container">
News & Events
</span>
</a>
<div aria-labelledby="ubcms-gen-33853671" class="topnav-submenu-container">
<ul class="submenu clearfix">
<li class="first">
<a aria-label="News & Events:News" href="/cas/math/news-events/news.html">
News
</a>
</li>
<li>
<a aria-label="News & Events:Events " href="/cas/math/news-events/calendar.html">
Events
</a>
<div class="topnav-submenu-children-container list">
<ul class="submenu-children clearfix link-list">
<li class="first">
<a aria-label="Events :Class of 2021" href="/cas/math/people/alumni-friends/class-of-2021.html">
Class of 2021
</a>
</li>
<li class="last">
<a aria-label="Events :Class of 2020" href="/cas/math/people/alumni-friends/class-of-2020.html">
Class of 2020
</a>
</li>
</ul>
</div>
</li>
<li>
<a aria-label="News & Events:Myhill Lecture Series" href="/cas/math/news-events/myhill.html">
Myhill Lecture Series
</a>
<div class="topnav-submenu-children-container list">
<ul class="submenu-children clearfix link-list">
<li class="first">
<a aria-label="Myhill Lecture Series:Laura DeMarco, 2019" href="/cas/math/news-events/myhill/laura-demarco.html">
Laura DeMarco, 2019
</a>
</li>
<li>
<a aria-label="Myhill Lecture Series:Mark Newman, 2018" href="/cas/math/news-events/myhill/mark-newman.html">
Mark Newman, 2018
</a>
</li>
<li>
<a aria-label="Myhill Lecture Series:Guoliang Yu, 2017" href="/cas/math/news-events/myhill/guoliangyu.html">
Guoliang Yu, 2017
</a>
</li>
<li>
<a aria-label="Myhill Lecture Series:Gopal Prasad, 2016" href="/cas/math/news-events/myhill/gopal-prasad.html">
Gopal Prasad, 2016
</a>
</li>
<li>
<a aria-label="Myhill Lecture Series:Ciprian Manolescu, 2015" href="/cas/math/news-events/myhill/ciprian-manolescu.html">
Ciprian Manolescu, 2015
</a>
</li>
<li>
<a aria-label="Myhill Lecture Series:Percy A. Deift, 2014" href="/cas/math/news-events/myhill/percy-deift.html">
Percy A. Deift, 2014
</a>
</li>
<li class="last">
<a aria-label="Myhill Lecture Series:Peter Sarnak, 2013" href="/cas/math/news-events/myhill/peter-sarnak.html">
Peter Sarnak, 2013
</a>
</li>
</ul>
</div>
</li>
<li>
<a aria-label="News & Events:NERCCS 2020" href="/cas/math/news-events/nerccs-2020.html">
NERCCS 2020
</a>
</li>
<li>
<a aria-label="News & Events:News & Events Archive" href="/cas/math/news-events/archives.html">
News & Events Archive
</a>
</li>
<li class="last">
<a aria-label="News & Events:Visiting UB" href="/cas/math/news-events/visiting.html">
Visiting UB
</a>
</li>
</ul>
<div class="relatedLinks relatedlinksreference reference parbase">
</div>
</div>
</li>
</ul>
</div>
<div class="right">
<div class="search">
<!--noindex-->
<div class="search-menu" tabindex="0">
<div class="search-label">
Search
</div>
<!-- Uses appendAround.js script to transfer this search form to mobile nav menu via data-set attribute. -->
<div class="search-content" data-set="mobile-search">
<form action="/cas/math/searchresults.html" class="search-form" method="GET" onsubmit="return this.q.value != ''">
<div class="search-container" role="search">
<input aria-label="Search" autocomplete="off" class="search-input" id="ubcms-gen-33853673" name="q" placeholder="Search" type="text"/>
<button aria-label="Search" class="search-submit" type="submit" value="Search">
</button>
</div>
</form>
</div>
</div>
<!--endnoindex-->
</div>
<div class="audiencenav list parbase">
<div class="audiencenav-wrapper" tabindex="0">
<div class="label">
Info For
</div>
<ul>
<li>
<a href="/cas/math/information-for-students.html" onblur="jQuery(this).parents('.audiencenav-wrapper').removeClass('hover')" onfocus="jQuery(this).parents('.audiencenav-wrapper').addClass('hover')">
Current Students
</a>
</li>
<li>
<a href="/cas/math/ug/undergraduate-programs.html" onblur="jQuery(this).parents('.audiencenav-wrapper').removeClass('hover')" onfocus="jQuery(this).parents('.audiencenav-wrapper').addClass('hover')">
Future Undergraduate Students
</a>
</li>
<li>
<a href="/cas/math/grad.html" onblur="jQuery(this).parents('.audiencenav-wrapper').removeClass('hover')" onfocus="jQuery(this).parents('.audiencenav-wrapper').addClass('hover')">
Future Graduate Students
</a>
</li>
<li>
<a href="/cas/math/information-for-faculty-staff.html" onblur="jQuery(this).parents('.audiencenav-wrapper').removeClass('hover')" onfocus="jQuery(this).parents('.audiencenav-wrapper').addClass('hover')">
Faculty & Staff
</a>
</li>
<li>
<a href="/cas/math/people/alumni-friends.html" onblur="jQuery(this).parents('.audiencenav-wrapper').removeClass('hover')" onfocus="jQuery(this).parents('.audiencenav-wrapper').addClass('hover')">
Alumni & Friends
</a>
</li>
<li>
<a href="http://www.buffalo.edu/inclusion/resources/IXResources.html" onblur="jQuery(this).parents('.audiencenav-wrapper').removeClass('hover')" onfocus="jQuery(this).parents('.audiencenav-wrapper').addClass('hover')">
Diversity, Equity, and Inclusive Excellence Resources
</a>
</li>
</ul>
</div>
</div>
</div>
</nav>
<script>
$(".topnav").accessibleDropDown();
</script>
</div>
</div>
</div>
<div contenttreeid="innerheader" contenttreestatus="Not published" style="display:none;">
</div>
</div>
</header>
<div class="two-column clearfix" id="columns">
<div class="columns-bg columns-bg-1">
<div class="columns-bg columns-bg-2">
<div class="columns-bg columns-bg-3">
<div class="columns-bg columns-bg-4">
<div id="left">
<div class="leftnav">
<nav aria-label="section navigation" class="inner">
<div class="title">
<a href="/cas/math/people.html">
<span class="title">
People
</span>
</a>
</div>
<ul class="menu nav-level-1">
<li class="first">
<a aria-label="People:Faculty" href="/cas/math/people/faculty.html">
Faculty
</a>
</li>
<li>
<a aria-label="People:Staff" href="/cas/math/people/staff_directory.html">
Staff
</a>
</li>
<li>
<a aria-label="People:Emeriti Faculty" href="/cas/math/people/emeriti.html">
Emeriti Faculty
</a>
</li>
<li>
<a aria-label="People:Instructors" href="/cas/math/people/instructors.html">
Instructors
</a>
</li>
<li>
<a aria-label="People:Visiting Scholars" href="/cas/math/people/visiting-scholars.html">
Visiting Scholars
</a>
</li>
<li class="active-trail">
<span>
<a aria-label="People:Graduate Student Directory" class="active" href="/cas/math/people/grad-directory.html">
Graduate Student Directory
</a>
</span>
</li>
<li class="last expand-submenu">
<a aria-label="People:Alumni" href="/cas/math/people/alumni-friends.html">
Alumni
</a>
<ul class="menu nav-level-2">
<li class="first expand-submenu">
<a aria-label="Alumni:Class of 2021" href="/cas/math/people/alumni-friends/class-of-2021.html">
Class of 2021
</a>
</li>
<li class="expand-submenu">
<a aria-label="Alumni:Class of 2020" href="/cas/math/people/alumni-friends/class-of-2020.html">
Class of 2020
</a>
</li>
<li class="last expand-submenu">
<a aria-label="Alumni:PhD Recipients, 2010 to Present" href="/cas/math/grad/PhD-recipients.html">
PhD Recipients, 2010 to Present
</a>
</li>
</ul>
</li>
</ul>
<div class="relatedLinks relatedlinksreference reference parbase">
</div>
</nav>
</div>
<div class="mobile-left-col hide-in-narrow" data-set="mobile-center-bottom-or-right-top">
<div class="leftcol parsys iparsys" role="complementary">
<div class="section">
<div class="new">
</div>
</div>
<div class="iparys_inherited">
<div class="leftcol iparsys parsys">
<div class="flexmodule imagebase section">
<div class="flexmodule-inner" id="ubcms-gen-33853676">
<div class="title">
<h2>
Diversity, Equity, and Inclusive Excellence Resources
</h2>
</div>
<div class="teaser teaser-block flexmodule-style flexmodule-style-largeimg">
<div class="teaser-inner">
<div class="teaser-images">
<div class="teaser-image">
<a href="http://www.buffalo.edu/inclusion/resources/IXResources.html" target="_blank">
<noscript>
<picture contenttreeid="flexmodule" contenttreestatus="Not published">
<source media="(max-width: 568px)" srcset="/content/cas/math/people/jcr:content/leftcol/flexmodule.img.448.280.m.q50.png/1607108331977.png, /content/cas/math/people/jcr:content/leftcol/flexmodule.img.576.361.m.q50.png/1607108331977.png 2x" type="image/png">
<source media="(max-width: 720px)" srcset="/content/cas/math/people/jcr:content/leftcol/flexmodule.img.688.431.q80.png/1607108331977.png" type="image/png">
<source srcset="/content/cas/math/people/jcr:content/leftcol/flexmodule.img.209.131.png/1607108331977.png, /content/cas/math/people/jcr:content/leftcol/flexmodule.img.418.262.q65.png/1607108331977.png 2x" type="image/png">
<img alt="Diversity, Equity, and Inclusive Excellence Resources. " class="img-209 img-209x131 cq-dd-image" height="131" src="/content/cas/math/people/_jcr_content/leftcol/flexmodule.img.209.131.png/1607108331977.png" srcset="/content/cas/math/people/jcr:content/leftcol/flexmodule.img.418.262.q65.png/1607108331977.png 2x" width="209"/>
</source>
</source>
</source>
</picture>
</noscript>
<picture class="no-display" contenttreeid="flexmodule" contenttreestatus="Not published">
<source data-srcset="/content/cas/math/people/jcr:content/leftcol/flexmodule.img.448.280.m.q50.png/1607108331977.png, /content/cas/math/people/jcr:content/leftcol/flexmodule.img.576.361.m.q50.png/1607108331977.png 2x" media="(max-width: 568px)" type="image/png">
<source data-srcset="/content/cas/math/people/jcr:content/leftcol/flexmodule.img.688.431.q80.png/1607108331977.png" media="(max-width: 720px)" type="image/png">
<source data-srcset="/content/cas/math/people/jcr:content/leftcol/flexmodule.img.209.131.png/1607108331977.png, /content/cas/math/people/jcr:content/leftcol/flexmodule.img.418.262.q65.png/1607108331977.png 2x" type="image/png">
<img alt="Diversity, Equity, and Inclusive Excellence Resources. " class="img-209 img-209x131 cq-dd-image lazyload" data-src="/content/cas/math/people/jcr%3acontent/leftcol/flexmodule.img.209.131.png/1607108331977.png" data-srcset="/content/cas/math/people/jcr:content/leftcol/flexmodule.img.418.262.q65.png/1607108331977.png 2x" height="131" width="209"/>
</source>
</source>
</source>
</picture>
<script>
jQuery('picture.no-display').removeClass('no-display');
</script>
</a>
</div>
</div>
<div class="teaser-content">
<div class="teaser-body">
<p>
UB is committed to achieving inclusive excellence in a deliberate, intentional and coordinated fashion, embedding it in every aspect of our operations. We aspire to foster a healthy, productive, ethical, fair, and affirming campus community to allow all students, faculty and staff to thrive and realize their full potential.
<br/>
</p>
</div>
<div class="teaser-links">
<div class="list">
<div data-columnize-row="1" id="ubcms-gen-33853677">
<ul class="link-list" data-columnize="1">
<li>
<span class="teaser teaser-inline">
<a href="http://www.buffalo.edu/equity/promoting-equal-employment-opportunity-and-diversity.html">
<span class="teaser-inner">
<!--noindex-->
<span class="teaser-date">
</span>
<!--endnoindex-->
<span class="teaser-title">
Promoting Equal Employment Opportunity and Diversity
</span>
</span>
</a>
</span>
</li>
<li>
<span class="teaser teaser-inline">
<a href="http://www.buffalo.edu/inclusion/resources/IXResources.html">
<span class="teaser-inner">
<!--noindex-->
<span class="teaser-date">
</span>
<!--endnoindex-->
<span class="teaser-title">
Inclusive Excellence Resources
</span>
</span>
</a>
</span>
</li>
<li>
<span class="teaser teaser-inline">
<a href="http://www.buffalo.edu/inclusion/resources/toolkits_trainings.html">
<span class="teaser-inner">
<!--noindex-->
<span class="teaser-date">
</span>
<!--endnoindex-->
<span class="teaser-title">
Toolkits
</span>
</span>
</a>
</span>
</li>
<li>
<span class="teaser teaser-inline">
<a href="https://www.buffalo.edu/studentlife/who-we-are/departments/diversity.html">
<span class="teaser-inner">
<!--noindex-->
<span class="teaser-date">
</span>
<!--endnoindex-->
<span class="teaser-title">
UB Intercultural and Diversity Center
</span>
</span>
</a>
</span>
</li>
<li>
<span class="teaser teaser-inline">
<a href="https://www.buffalo.edu/diversity-innovation.html">
<span class="teaser-inner">
<!--noindex-->
<span class="teaser-date">
</span>
<!--endnoindex-->
<span class="teaser-title">
UB Center for Diversity Innovation
</span>
</span>
</a>
</span>
</li>
<li>
<span class="teaser teaser-inline">
<a href="http://www.buffalo.edu/equity/external-resources.html">
<span class="teaser-inner">
<!--noindex-->
<span class="teaser-date">
</span>
<!--endnoindex-->
<span class="teaser-title">
External Resources
</span>
</span>
</a>
</span>
</li>
</ul>
</div>
<div class="clearfix">
</div>
<script>
UBCMS.list.listlimit('ubcms\u002Dgen\u002D33853677', '100',
'100');
</script>
</div>
</div>
</div>
</div>
<div class="teaser-clear">
</div>
</div>
</div>
<div class="flexmodule-clear">
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<script>
(function() {
var $firstLeftIparsysInherited = $('#left .iparys_inherited').eq(0);
var $firstLeftIparsysSection = $('#left > .iparsys:first-child > .section:first-child');
var $mcbort = $('.mobile-center-bottom-or-right-top');
if ($firstLeftIparsysInherited.length && $firstLeftIparsysInherited.html().replace(/\s+|<\/?div\b[^>]*>/gi, '') === '')
$firstLeftIparsysInherited.addClass('empty');
if ($firstLeftIparsysSection.length && $firstLeftIparsysSection.html().replace(/\s+|<\/?div\b[^>]*>/gi, '') === '')
$firstLeftIparsysSection.addClass('empty');
if ($mcbort.length && $mcbort.html().replace(/\s+|<\/?div\b[^>]*>/gi, '') === '')
$mcbort.addClass('empty');
$('[role=complementary]').each(function() {
var $this = $(this);
if ($this.children().filter(':not(.empty)').filter(':not(:empty)').length === 0)
$this.removeAttr('role');
});
if ($('.leftcol[role=complementary]').length > 0 && $('#right[role=complementary]').length > 0) {
$('.leftcol[role=complementary]').attr('aria-label', 'left column');
$('#right[role=complementary]').attr('aria-label', 'right column');
}
})();
</script>
<div id="skip-to-content">
</div>
<div id="center" role="main">
<div class="mobile-content-top" data-set="content-top">
</div>
<div class="par parsys">
<div class="title section">
<h1 id="title" onpaste="onPasteFilterPlainText(event)">
Graduate Student Directory
</h1>
</div>
<div class="image-container image-container-680">
<div class="image border-hide">
<noscript>
<picture contenttreeid="image" contenttreestatus="Not published">
<source media="(max-width: 568px)" srcset="/content/cas/math/people/grad-directory/jcr:content/par/image.img.448.auto.m.q50.jpg/1629919606956.jpg, /content/cas/math/people/grad-directory/jcr:content/par/image.img.576.auto.m.q50.jpg/1629919606956.jpg 2x" type="image/jpeg">
<source media="(max-width: 720px)" srcset="/content/cas/math/people/grad-directory/jcr:content/par/image.img.688.auto.q80.jpg/1629919606956.jpg" type="image/jpeg">
<source srcset="/content/cas/math/people/grad-directory/jcr:content/par/image.img.680.auto.jpg/1629919606956.jpg, /content/cas/math/people/grad-directory/jcr:content/par/image.img.1360.auto.q65.jpg/1629919606956.jpg 2x" type="image/jpeg">
<img alt="1. " class="img-680 cq-dd-image" src="/content/cas/math/people/grad-directory/_jcr_content/par/image.img.680.auto.jpg/1629919606956.jpg" srcset="/content/cas/math/people/grad-directory/jcr:content/par/image.img.1360.auto.q65.jpg/1629919606956.jpg 2x" width="680"/>
</source>
</source>
</source>
</picture>
</noscript>
<picture class="no-display" contenttreeid="image" contenttreestatus="Not published">
<source data-srcset="/content/cas/math/people/grad-directory/jcr:content/par/image.img.448.auto.m.q50.jpg/1629919606956.jpg, /content/cas/math/people/grad-directory/jcr:content/par/image.img.576.auto.m.q50.jpg/1629919606956.jpg 2x" media="(max-width: 568px)" type="image/jpeg">
<source data-srcset="/content/cas/math/people/grad-directory/jcr:content/par/image.img.688.auto.q80.jpg/1629919606956.jpg" media="(max-width: 720px)" type="image/jpeg">
<source data-srcset="/content/cas/math/people/grad-directory/jcr:content/par/image.img.680.auto.jpg/1629919606956.jpg, /content/cas/math/people/grad-directory/jcr:content/par/image.img.1360.auto.q65.jpg/1629919606956.jpg 2x" type="image/jpeg">
<img alt="1. " class="img-680 cq-dd-image lazyload" data-src="/content/cas/math/people/grad-directory/jcr%3acontent/par/image.img.680.auto.jpg/1629919606956.jpg" data-srcset="/content/cas/math/people/grad-directory/jcr:content/par/image.img.1360.auto.q65.jpg/1629919606956.jpg 2x" width="680"/>
</source>
</source>
</source>
</picture>
<script>
jQuery('picture.no-display').removeClass('no-display');
</script>
<!-- <span data-sly-test="false" class="aria-hidden"></span> -->
</div>
</div>
<div class="title section">
<h2 id="top" onpaste="onPasteFilterPlainText(event)">
Mathematics Graduate Student Directory 2021-2022
</h2>
</div>
<div class="introtext text parbase section">
<p>
<a href="#letter_a">
A
</a>
|
<a href="#letter_b">
B
</a>
|
<a href="#letter_c">
C
</a>
|
<a href="#letter_d">
D
</a>
| E |
<a href="#letter_f">
F
</a>
|
<a href="#letter_g">
G
</a>
|
<a href="#letter_h">
H
</a>
| I |
<a href="#letter_j">
J
</a>
|
<a href="#letter_h">
K
</a>
|
<a href="#letter_l">
L
</a>
|
<a href="#letter_m">
M
</a>
|
<a href="#letter_n">
N
</a>
|
<a href="#letter_o">
O
</a>
|
<a href="#letter_p">
P
</a>
| Q |
<a href="#letter_r">
R
</a>
|
<a href="#letter_s">
S
</a>
|
<a href="#letter_t">
T
</a>
|
<a href="#letter_u">
U
</a>
|
<a href="#letter_v">
V
</a>
|
<a href="#letter_w">
W
</a>
|
<a href="#letter_x">
X
</a>
|
<a href="#letter_y">
Y
</a>
|
<a href="#letter_z">
Z
</a>
</p>
</div>
<div class="hr hrline" style="clear:left">
</div>
<div class="text parbase section">
<p>
<i>
Offices as listed are in the Mathematics Building, North Campus.
</i>
</p>
</div>
<div class="hr hrline" style="clear:left">
</div>
<div class="title section">
<h2 id="title_3" onpaste="onPasteFilterPlainText(event)">
A
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Abeya Ranasinghe Mudiyanselage, Asela V.
</b>
<br/>
Office: 138 Phone: 645-8823
<br/>
Email:
<a href="mailto:aselavir@buffalo.edu">
aselavir@buffalo.edu
</a>
</p>
<p>
<b>
Ahn, Min Woong
</b>
<br/>
Office: 126 Phone: 645-8816
<br/>
Email:
<a href="mailto:minwoong@buffalo.edu">
minwoong@buffalo.edu
</a>
</p>
<p>
<b>
Alegria, Linda
</b>
<br/>
Office: 138 Phone: 645-8823
<br/>
Email:
<a href="mailto:lindaale@buffalo.edu">
lindaale@buffalo.edu
</a>
<br/>
</p>
</div>
<div class="hr hrline" style="clear:left">
</div>
<div class="title section">
<h2 id="letter_b" onpaste="onPasteFilterPlainText(event)">
B
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Betz, Katherine
</b>
<br/>
Office: 132 Phone: 645-8820
<br/>
Email:
<a href="mailto:kbetz2@buffalo.edu">
kbetz2@buffalo.edu
</a>
</p>
<p>
<b>
Bhaumik, Jnanajyoti
</b>
<br/>
Office: 129 Mathematics Building
<br/>
Phone: 645-8817
<br/>
Email:
<a href="mailto:jnanajyo@buffalo.edu">
jnanajyo@buffalo.edu
</a>
</p>
<p>
</p>
</div>
<div class="calltoaction section">
<span class="teaser teaser-inline calltoaction-style-small">
<a href="#top">
<span class="teaser-inner">
<span class="teaser-title">
back to top
</span>
</span>
</a>
</span>
</div>
<div class="hr hrline" style="clear:left">
</div>
<div class="title section">
<h2 id="letter_c" onpaste="onPasteFilterPlainText(event)">
C
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Cain Charles
</b>
<br/>
Email:
<a href="mailto:ccain2@buffalo.edu">
ccain2@buffalo.edu
</a>
</p>
<p>
<b>
Casper, Michael
<br/>
</b>
Office: 222 Phone: 645-8779
<br/>
Email:
<a href="mailto:mjcasper@buffalo.edu">
mjcasper@buffalo.edu
</a>
</p>
<p>
<b>
Chang, Hong
<br/>
</b>
Office: 136 Phone: 645-8821
<br/>
Email:
<a href="mailto:hchang24@buffalo.edu">
hchang24@buffalo.edu
</a>
</p>
<p>
<b>
Chen, Yen-Lin
<br/>
</b>
Office: 125 Phone: 645-8815
<br/>
Email:
<a href="mailto:yenlinch@buffalo.edu">
yenlinch@buffalo.edu
</a>
<br/>
</p>
<p>
<b>
Cheuk, Ka Yue
<br/>
</b>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:kayueche@buffalo.edu">
kayueche@buffalo.edu
</a>
</p>
<p>
<b>
Cosgrove, Gage (Makenzie)
<br/>
</b>
Office: 139 Phone: 645-8824
<br/>
Email:
<a href="mailto:gagecosg@buffalo.edu">
gagecosg@buffalo.edu
</a>
</p>
<p>
</p>
</div>
<div class="hr hrline" style="clear:left">
</div>
<div class="calltoaction section">
<span class="teaser teaser-inline calltoaction-style-small">
<a href="#top">
<span class="teaser-inner">
<span class="teaser-title">
back to top
</span>
</span>
</a>
</span>
</div>
<div class="title section">
<h2 id="letter_d" onpaste="onPasteFilterPlainText(event)">
D
</h2>
</div>
<div class="title section">
<h2 id="letter_e" onpaste="onPasteFilterPlainText(event)">
E
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Engelhardt, Carolyn
<br/>
</b>
Email:
<a href="mailto:cengelha@buffalo.edu">
cengelha@buffalo.edu
</a>
<br/>
</p>
</div>
<div class="title section">
<h2 id="letter_f" onpaste="onPasteFilterPlainText(event)">
F
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Fonseca dos Reis, Elohim
<br/>
</b>
Office: 313 Phone: 645-8804
<br/>
Email:
<a href="mailto:elohimfo@buffalo.edu">
elohimfo@buffalo.edu
</a>
</p>
</div>
<div class="title section">
<h2 id="letter_g" onpaste="onPasteFilterPlainText(event)">
G
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Gkogkou, Aikaterini
</b>
<br/>
Office: 129 Phone: 645-8817
<br/>
Email:
<a href="mailto:agkogkou@buffalo.edu">
agkogkou@buffalo.edu
</a>
</p>
</div>
<div class="title section">
<h2 id="letter_h" onpaste="onPasteFilterPlainText(event)">
H
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Haverlick, Justin
<br/>
</b>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:jmhaverl@buffalo.edu">
jmhaverl@buffalo.edu
</a>
</p>
<p>
<b>
Herron, Amy
</b>
<br/>
Office: 135
<br/>
Email:
<a href="mailto:ajherron@buffalo.edu">
ajherron@buffalo.edu
</a>
</p>
<p>
<b>
Hovland, Seth
</b>
<br/>
Office: 130 Phone: 645-8818
<br/>
Email:
<a href="mailto:sethhovl@buffalo.edu">
sethhovl@buffalo.edu
</a>
</p>
<p>
<b>
Hung, Tsz Fun
</b>
<br/>
Office: 137 Phone: 645-8822
<br/>
Email:
<a href="mailto:tszfunhu@buffalo.edu">
tszfunhu@buffalo.edu
</a>
</p>
<p>
<b>
Hutchings, Raymond
</b>
<br/>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:rhhutchi@buffalo.edu">
rhhutchi@buffalo.edu
</a>
</p>
<p>
<b>
Huynh, Bao
<br/>
</b>
Office: 131 Phone: 645-8819
<br/>
Email:
<a href="mailto:baohuynh@buffalo.edu">
baohuynh@buffalo.edu
</a>
</p>
</div>
<div class="calltoaction section">
<span class="teaser teaser-inline calltoaction-style-small">
<a href="#top">
<span class="teaser-inner">
<span class="teaser-title">
back to top
</span>
</span>
</a>
</span>
</div>
<div class="title section">
<h2 id="letter_j" onpaste="onPasteFilterPlainText(event)">
J
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Jeong, Myeongjin
<br/>
</b>
Office: 244
<br/>
Email:
<a href="mailto:mjeong31@buffalo.edu">
mjeong31@buffalo.edu
</a>
</p>
<p>
<b>
Jones, Raymond
</b>
<br/>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:rpjones2@buffalo.edu">
rpjones2@buffalo.edu
</a>
</p>
</div>
<div class="title section">
<h2 id="letter_k" onpaste="onPasteFilterPlainText(event)">
K
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Kilic, Bengier Ulgen
<br/>
</b>
Office: 106 Phone: 645-8763
<br/>
Email:
<a href="mailto:bengieru@buffalo.edu">
bengieru@buffalo.edu
</a>
</p>
<p>
<b>
Kim, Jiseong
<br/>
</b>
Office: 125 Phone: 645-8815
<br/>
Email:
<a href="mailto:jiseongk@buffalo.edu">
jiseongk@buffalo.edu
</a>
</p>
<p>
<b>
Kireyev, Dmitri
</b>
<br/>
Office: 129 Phone: 645-8817
<br/>
Email:
<a href="mailto:dmitriki@buffalo.edu">
dmitriki@buffalo.edu
</a>
<br/>
</p>
</div>
<div class="calltoaction section">
<span class="teaser teaser-inline calltoaction-style-small">
<a href="#top">
<span class="teaser-inner">
<span class="teaser-title">
back to top
</span>
</span>
</a>
</span>
</div>
<div class="title section">
<h2 id="letter_l" onpaste="onPasteFilterPlainText(event)">
L
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Le, Minh Quang
</b>
<br/>
Office: 131 Phone: 645-8819
<br/>
Email:
<a href="mailto:minhquan@buffalo.edu">
minhquan@buffalo.edu
</a>
</p>
<p>
<b>
Leonard, Dakota
</b>
<br/>
Office: 131 Phone: 645-8819
<br/>
Email:
<a href="mailto:dl42@buffalo.edu">
dl42@buffalo.edu
</a>
</p>
<p>
<b>
Liao, Chang-Chih
</b>
<br/>
Office: 138 Phone: 645-8823
<br/>
Email:
<a href="mailto:cliao9@buffalo.edu">
cliao9@buffalo.edu
</a>
</p>
<p>
<b>
Liao, Yanzhan
</b>
<br/>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:yanzhanl@buffalo.edu">
yanzhanl@buffalo.edu
</a>
</p>
<p>
<b>
Liu, Ruodan
</b>
<br/>
Office: 135 Phone: 645-8832
<br/>
Email:
<a href="mailto:rliu8@buffalo.edu">
rliu8@buffalo.edu
</a>
</p>
<p>
<b>
Liu,Tianmou
<br/>
</b>
Office: 136 Phone: 645-8821
<br/>
Email:
<a href="mailto:tianmoul@buffalo.edu">
tianmoul@buffalo.edu
</a>
</p>
<p>
<b>
Liu, Yuan
</b>
<br/>
Office: 135 Phone: 645-8832
<br/>
Email:
<a href="mailto:yuanliu@buffalo.edu">
yuanliu@buffalo.edu
</a>
</p>
<p>
</p>
</div>
<div class="calltoaction section">
<span class="teaser teaser-inline calltoaction-style-small">
<a href="#top">
<span class="teaser-inner">
<span class="teaser-title">
back to top
</span>
</span>
</a>
</span>
</div>
<div class="title section">
<h2 id="letter_m" onpaste="onPasteFilterPlainText(event)">
M
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Ma, Ning
<br/>
</b>
Office: 125 Phone: 645-8815
<br/>
Email:
<a href="mailto:nma22@buffalo.edu">
nma22@buffalo.edu
</a>
<br/>
</p>
<p>
<b>
Ma, Renda
<br/>
</b>
Office: 125 Phone: 645-8815
<br/>
Email:
<a href="mailto:rendama@buffalo.edu">
rendama@buffalo.edu
</a>
</p>
<p>
<b>
Ma, Yuqing
</b>
<br/>
Office: 138 Phone: 645-8823
<br/>
Email:
<a href="mailto:yuqingma@buffalo.edu">
yuqingma@buffalo.edu
</a>
</p>
<p>
<b>
Meng, Lingqi
<br/>
</b>
Office: 130 Phone: 645-8818
<br/>
Email:
<a href="mailto:lingqime@buffalo.edu">
lingqime@buffalo.edu
</a>
</p>
<p>
<b>
Meyer, Drew
</b>
<br/>
Office: 137 Phone: 645-8822
<br/>
Email:
<a href="mailto:drewmeye@buffalo.edu">
drewmeye@buffalo.edu
</a>
</p>
<p>
<b>
Montoro, Michael
<br/>
</b>
Office: 126 Phone: 645-8816
<br/>
Email:
<a href="mailto:mnmontor@buffalo.edu">
mnmontor@buffalo.edu
</a>
</p>
<p>
<b>
Moore, Robert
</b>
<br/>
Office: 139 Phone: 645-8824
<br/>
Email:
<a href="mailto:rcmoore@buffalo.edu">
rcmoore@buffalo.edu
</a>
<br/>
</p>
</div>
<div class="title section">
<h2 id="letter_n" onpaste="onPasteFilterPlainText(event)">
N
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Nguyen, Khanh Truong
</b>
<br/>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:kn55@buffalo.edu">
kn55@buffalo.edu
</a>
</p>
</div>
<div class="calltoaction section">
<span class="teaser teaser-inline calltoaction-style-small">
<a href="#top">
<span class="teaser-inner">
<span class="teaser-title">
back to top
</span>
</span>
</a>
</span>
</div>
<div class="title section">
<h2 id="letter_o" onpaste="onPasteFilterPlainText(event)">
O
</h2>
</div>
<div class="title section">
<h2 id="letter_p" onpaste="onPasteFilterPlainText(event)">
P
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Peng, Jun
</b>
<br/>
Office: 139 Phone: 645-8824
<b>
<br/>
</b>
Email:
<a href="mailto:jpeng3@buffalo.edu">
jpeng3@buffalo.edu
</a>
</p>
<p>
<b>
Poste, Alex
</b>
<br/>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:apposte@buffalo.edu">
apposte@buffalo.edu
</a>
</p>
<p>
</p>
</div>
<div class="title section">
<h2 id="letter_r" onpaste="onPasteFilterPlainText(event)">
R
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Rantanen, Matthew
<br/>
</b>
Office: 130 Phone: 645-8818
<br/>
Email:
<a href="mailto:mattrant@buffalo.edu">
mattrant@buffalo.edu
</a>
</p>
<p>
<b>
Rele, Adhish
</b>
<br/>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:adhishpr@buffalo.edu">
adhishpr@buffalo.edu
</a>
</p>
<p>
<b>
Russell, Madison
</b>
<br/>
Office: 129 Phone: 645-8817
<br/>
Email:
<a href="mailto:merussel@buffalo.edu">
merussel@buffalo.edu
</a>
</p>
<p>
<b>
Rozwood, Bud
<br/>
</b>
Office: 125 Phone: 645-8815
<b>
<br/>
</b>
Email:
<a href="mailto:budrozwo@buffalo.edu">
budrozwo@buffalo.edu
</a>
</p>
</div>
<div class="title section">
<h2 id="letter_s" onpaste="onPasteFilterPlainText(event)">
S
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Sarkar, Sayantan
<br/>
</b>
Office: 125 Phone: 645-8815
<br/>
Email:
<a href="mailto:sayantan@buffalo.edu">
sayantan@buffalo.edu
</a>
</p>
<p>
<b>
Sharma, Abhishek
<br/>
</b>
Office: 126 Phone: 645-8816
<br/>
Email: aks28
<a href="mailto:sayantan@buffalo.edu">
@buffalo.edu
</a>
</p>
<p>
<b>
Sicuso, Luca
</b>
<br/>
Office: 131 Phone: 645-8819
<br/>
Email:
<a href="mailto:lucasicu@buffalo.edu">
lucasicu@buffalo.edu
</a>
</p>
<p>
<b>
Solanki, Deepisha
</b>
<br/>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:deepisha@buffalo.edu">
deepisha@buffalo.edu
</a>
</p>
<p>
<b>
Som, Bratati
<br/>
</b>
Office: 132 Phone: 645-8820
<br/>
Email:
<a href="mailto:bratatis@buffalo.edu">
bratatis@buffalo.edu
</a>
</p>
<p>
<b>
Song, Zhao
<br/>
</b>
Office: 131 Phone: 645-8819
<br/>
Email:
<a href="mailto:zhaosong@buffalo.edu">
zhaosong@buffalo.edu
</a>
</p>
<p>
<b>
Sullivan, Mark
</b>
<br/>
Office: 136 Phone: 645-8821
<br/>
Email:
<a href="mailto:marksull@buffalo.edu">
marksull@buffalo.edu
</a>
</p>
<p>
<b>
Sun, Yuxun
</b>
<br/>
Office: 137 Phone: 645-8822
<br/>
Email:
<a href="mailto:yuxunsun@buffalo.edu">
yuxunsun@buffalo.edu
</a>
</p>
</div>
<div class="title section">
<h2 id="letter_t" onpaste="onPasteFilterPlainText(event)">
T
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Thongprayoon, Chanon
</b>
<br/>
Office: 125 Phone: 645-8815
<br/>
Email:
<a href="mailto:chanonth@buffalo.edu">
chanonth@buffalo.edu
</a>
<br/>
<br/>
<br/>
</p>
</div>
<div class="calltoaction section">
<span class="teaser teaser-inline calltoaction-style-small">
<a href="#top">
<span class="teaser-inner">
<span class="teaser-title">
back to top
</span>
</span>
</a>
</span>
</div>
<div class="title section">
<h2 id="letter_u" onpaste="onPasteFilterPlainText(event)">
U
</h2>
</div>
<div class="title section">
<h2 id="letter_v" onpaste="onPasteFilterPlainText(event)">
V
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Vadnere, Arya Abhijeet
</b>
<br/>
Office: 132 Mathematics Building
<br/>
Phone: 645-8820
<br/>
Email:
<a href="mailto:aryaabhi@buffalo.edu">
aryaabhi@buffalo.edu
</a>
</p>
<p>
<b>
Vinal, Gregory
<br/>
</b>
Office: 126 Phone: 645-8816
<br/>
Email:
<a href="mailto:chanonth@buffalo.edu">
gregoryv@buffalo.edu
</a>
</p>
<p>
</p>
</div>
<div class="title section">
<h2 id="letter_w" onpaste="onPasteFilterPlainText(event)">
W
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Wang, Daxun
<br/>
</b>
Office: 141 Phone: 645-8825
<br/>
Email:
<a href="mailto:daxunwan@buffalo.edu">
daxunwan@buffalo.edu
</a>
</p>
<p>
<b>
Wang, Shiruo
<br/>
</b>
Office: 125 Phone: 645-8815
<br/>
Email:
<a href="mailto:bwang32@buffalo.edu">
shiruowa@buffalo.edu
</a>
</p>
</div>
<div class="calltoaction section">
<span class="teaser teaser-inline calltoaction-style-small">
<a href="#top">
<span class="teaser-inner">
<span class="teaser-title">
back to top
</span>
</span>
</a>
</span>
</div>
<div class="title section">
<h2 id="letter_y" onpaste="onPasteFilterPlainText(event)">
Y
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Yuan, Cheng
</b>
<br/>
Office: 137 Phone: 645-8822
<br/>
Email:
<a href="mailto:chengyua@buffalo.edu">
chengyua@buffalo.edu
</a>
</p>
</div>
<div class="title section">
<h2 id="letter_z" onpaste="onPasteFilterPlainText(event)">
Z
</h2>
</div>
<div class="text parbase section">
<p>
<b>
Zhang, Baoming
</b>
<br/>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:baomingz@buffalo.edu">
baomingz@buffalo.edu
</a>
</p>
<p>
<b>
Zhao, Bojun
</b>
<br/>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:bojunzha@buffalo.edu">
bojunzha@buffalo.edu
</a>
</p>
<p>
<b>
Ziegler, Cameron
</b>
<br/>
Office: 140 Phone: 645-8825
<br/>
Email:
<a href="mailto:cz22@buffalo.edu">
cz22@buffalo.edu
</a>
</p>
</div>
<div class="calltoaction section">
<span class="teaser teaser-inline calltoaction-style-small">
<a href="#top">
<span class="teaser-inner">
<span class="teaser-title">
back to top
</span>
</span>
</a>
</span>
</div>
</div>
<div class="mobile-content-bottom" data-set="content-bottom">
</div>
<div class="mobile-center-or-right-bottom" data-set="center-or-right-bottom">
</div>
<div class="mobile-center-bottom-or-right-top" data-set="mobile-center-bottom-or-right-top">
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<footer>
<div class="footer inheritedreference reference parbase">
<div class="footerconfigpage contentpage page basicpage">
<div class="par parsys">
<div class="htmlsnippet section">
<div>
<style type="text/css">
@only screen and (max-width: 720px){
.simplefooter .simplefootercontents > .copyright {
clear: both;
position: relative;
top: 7px;
}
}
</style>
</div>
</div>
<div class="fatfooter section">
<div class="footer-mode-simple clearfix">
<a class="ub-logo-link" href="//www.buffalo.edu/">
<img alt="University at Buffalo The State University of New York - 175 Years: 1846-2021" class="ub-logo" src="/v-e541efb31faa2518c910054a542e1234/etc.clientlibs/wci/components/block/fatfooter/clientlibs/resources/ub-logo-175-years.png" width="400"/>
</a>
<div class="footer-columns footer-columns-1">
<div class="footer-column footer-column-1">
<div class="col1 parsys">
<div class="title section">
<h2 id="title-1" onpaste="onPasteFilterPlainText(event)">
<a href="/cas/math.html">
Department of Mathematics
</a>
</h2>
</div>
<div class="text parbase section">
<p>
244 Mathematics Building
<br/>
Buffalo, NY 14260-2900
<br/>
Phone: (716) 645-6284
<br/>
Fax: (716) 645-5039
</p>
</div>
<div class="reference parbase section">
<div class="unstructuredpage page basicpage">
<div class="par parsys">
<div class="hr hrline" style="clear:left">
</div>
<div class="captiontext text parbase section">
<p>
<b>
About Our Photos and Videos:
</b>
Some photos or videos that appear on this site may have been taken prior to the COVID-19 pandemic and therefore may not accurately reflect current operations or adherence to
<a href="https://www.buffalo.edu/coronavirus/health-and-safety.html" target="_blank">
UB’s Health and Safety Guidelines
</a>
.
<br/>
</p>
</div>
</div>
</div>
<div contenttreeid="reference-1" contenttreestatus="Not published" style="display:none;">
</div>
</div>
</div>
</div>
</div>
<div class="copyright">
<span class="copy">
</span>
<script>
jQuery(".copyright .copy").html("© " + (new Date()).getFullYear());
</script>
<a href="//www.buffalo.edu/">
University at Buffalo
</a>
. All rights reserved. |
<a href="//www.buffalo.edu/administrative-services/policy1/ub-policy-lib/privacy.html">
Privacy
</a>
|
<a href="//www.buffalo.edu/access/about-us/contact-us.html">
Accessibility
</a>
</div>
</div>
</div>
<div class="htmlsnippet section">
<div>
<!-- Global site tag (gtag.js) - Google Analytics -->
<script async="" src="https://www.googletagmanager.com/gtag/js?id=UA-127757988-27">
</script>
<script>
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', 'UA-127757988-27');
</script>
</div>
</div>
</div>
</div>
<div contenttreeid="footer" contenttreestatus="Not published" style="display:none;">
</div>
</div>
</footer>
</body>
</html>
The main content of the web page consists of contact information of math graduate students. Our goal will be to retrieve names of the students listed on the page. Looking at the code above we can see that each student listing is enclosed in the HTML paragraph tag <p>....</p>
, e.g:
<p>
<b>
Smith, John
<br/>
</b>
Office: 118 Phone: 645-8880
<br/>
Email: jsmith123@buffalo.edu
</p>
We can use the find_all()
method to search for all HTML tags of a given kind. For example, soup.find_all('p')
returns a list of all paragraph tags:
[7]:
ptags = soup.find_all('p')
ptags[:10]
[7]:
[<p><b>COVID-19 UPDATES • 3/4/2022</b></p>,
<p>UB is committed to achieving inclusive excellence in a deliberate, intentional and coordinated fashion, embedding it in every aspect of our operations. We aspire to foster a healthy, productive, ethical, fair, and affirming campus community to allow all students, faculty and staff to thrive and realize their full potential. <br/></p>,
<p><a href="#letter_a">A</a> | <a href="#letter_b">B</a> | <a href="#letter_c">C</a> | <a href="#letter_d">D</a> | E | <a href="#letter_f">F</a> | <a href="#letter_g">G</a> | <a href="#letter_h">H</a> | I | <a href="#letter_j">J</a> | <a href="#letter_h">K</a> | <a href="#letter_l">L</a> | <a href="#letter_m">M</a> | <a href="#letter_n">N</a> | <a href="#letter_o">O</a> | <a href="#letter_p">P</a> | Q | <a href="#letter_r">R</a> | <a href="#letter_s">S</a> | <a href="#letter_t">T</a> | <a href="#letter_u">U</a> | <a href="#letter_v">V</a> | <a href="#letter_w">W</a> | <a href="#letter_x">X</a> | <a href="#letter_y">Y</a> | <a href="#letter_z">Z</a></p>,
<p><i>Offices as listed are in the Mathematics Building, North Campus.</i></p>,
<p><b>Abeya Ranasinghe Mudiyanselage, Asela V.</b><br/> Office: 138 Phone: 645-8823<br/> Email: <a href="mailto:aselavir@buffalo.edu">aselavir@buffalo.edu</a></p>,
<p><b>Ahn, Min Woong</b><br/> Office: 126 Phone: 645-8816<br/> Email: <a href="mailto:minwoong@buffalo.edu">minwoong@buffalo.edu</a></p>,
<p><b>Alegria, Linda</b><br/> Office: 138 Phone: 645-8823<br/> Email: <a href="mailto:lindaale@buffalo.edu">lindaale@buffalo.edu</a><br/></p>,
<p><b>Betz, Katherine</b><br/> Office: 132 Phone: 645-8820<br/> Email: <a href="mailto:kbetz2@buffalo.edu">kbetz2@buffalo.edu</a></p>,
<p><b>Bhaumik, Jnanajyoti</b><br/> Office: 129 Mathematics Building<br/> Phone: 645-8817<br/> Email: <a href="mailto:jnanajyo@buffalo.edu">jnanajyo@buffalo.edu</a></p>,
<p> </p>]
Some items on the list (e.g. the first one) do not contain relevant information, but most of them are paragraphs with student data:
[10]:
ptags[11]
[10]:
<p><b>Casper, Michael<br/> </b> Office: 222 Phone: 645-8779<br/> Email: <a href="mailto:mjcasper@buffalo.edu">mjcasper@buffalo.edu</a></p>
Inside a paragraph tag the name of a student is enclosed in the tag <b>....</b>
indicating boldface text. We could use the find_all()
method again to search for boldface tags in a paragraph, but in this example we can use the find()
method as well. It returns the first occurrence of a given tag:
[11]:
print(ptags[11].find('b'))
<b>Casper, Michael<br/> </b>
The method find()
returns None
if the specified tag is not found. We can use this to filter out paragraphs which do not contain student names:
[13]:
# this paragraph gives the address of the Math Department
ptags[-2]
[13]:
<p>244 Mathematics Building<br/> Buffalo, NY 14260-2900<br/> Phone: (716) 645-6284<br/> Fax: (716) 645-5039</p>
[15]:
print(ptags[-2].find('b'))
None
The get_text()
method returns text contained in a specified HTML element:
[16]:
name = ptags[11].find('b')
name.get_text()
[16]:
'Casper, Michael '
Applying this to the list of paragraph tags with student data, we can extract names of all students:
[20]:
for p in ptags[:10]:
name = p.find('b')
if name is not None: # skip paragraphs with no b-tags
print(name.get_text())
Abeya Ranasinghe Mudiyanselage, Asela V.
Ahn, Min Woong
Alegria, Linda
Betz, Katherine
Bhaumik, Jnanajyoti
Links¶
Links in HTML documents are defined by the <a>
tag. For example:
<a href="https://www.buffalo.edu">University at Buffalo</a>
will be displayed in a web browser as University at Buffalo. The href
attribute specifies the address of the link destination, and the text between <a>
and </a>
is the visible text of the link.
Using Beautiful Soup we find links in the graduate student directory web page:
[21]:
grad_links = soup.find_all('a')
grad_links[:10] # show the first 10 links
[21]:
[<a href="#skip-to-content" id="skip-to-content-link">Skip to Content</a>,
<a href="http://arts-sciences.buffalo.edu/">College of Arts and Sciences</a>,
<a href="//www.buffalo.edu">UB Home</a>,
<a href="//www.buffalo.edu/maps">Maps</a>,
<a href="//www.buffalo.edu/directory/">UB Directory</a>,
<a href="//www.buffalo.edu"> <i class="icon icon-ub-logo"></i><span class="ada-hidden">University at Buffalo</span> <span class="logo"> <img alt="" class="black" height="20" src="/v-be9166b6b4a1ea7e5771e2eba1d410cf/etc.clientlibs/wci/components/block/header/clientlibs/resources/ub-logo-black.png" width="181"/> </span> </a>,
<a class="title" href="/cas/math.html">Department of Mathematics</a>,
<a href="http://www.buffalo.edu/ub_admissions/apply-now.html" target="_blank"> Apply to UB </a>,
<a href="http://www.buffalo.edu/cas/math/about-us/contact-us.html"> Contact Us </a>,
<a href="https://ubfoundation.buffalo.edu/giving/?gift_allocation=01-3-0-01240" target="_blank"> Support UB Math </a>]
Beautiful Soup lets us easily access attributes of any tag:
[22]:
link = grad_links[7]
link
[22]:
<a href="http://www.buffalo.edu/ub_admissions/apply-now.html" target="_blank"> Apply to UB </a>
[23]:
link.attrs # get a dictionary of attributes of a tag
[23]:
{'href': 'http://www.buffalo.edu/ub_admissions/apply-now.html',
'target': '_blank'}
[24]:
link['href'] # get the value of the 'href' attribute
[24]:
'http://www.buffalo.edu/ub_admissions/apply-now.html'
Link formats¶
Notice that links addresses can be given in several different formats.
An absolute link, with the whole address of the destination:
[25]:
grad_links[1]
[25]:
<a href="http://arts-sciences.buffalo.edu/">College of Arts and Sciences</a>
Also an absolute link, but the without the http:
part:
[26]:
grad_links[2]
[26]:
<a href="//www.buffalo.edu">UB Home</a>
A local link, omitting the http://www.buffalo.edu
part:
[27]:
grad_links[6]
[27]:
<a class="title" href="/cas/math.html">Department of Mathematics</a>
An anchor, which links to a specific part of the same page where the link is located. The full address would be http://www.buffalo.edu/cas/math/people/grad-directory.html#letter_a
:
[32]:
grad_links[118]
[32]:
<a href="#letter_a">A</a>
Images¶
Images in HTML documents are defined using the <img>
tag:
[61]:
image = soup.find_all('img')
img = image[1]
img
[61]:
<img alt="Diversity, Equity, and Inclusive Excellence Resources. " class="img-209 img-209x131 cq-dd-image" height="131" src="/content/cas/math/people/_jcr_content/leftcol/flexmodule.img.209.131.png/1607108331977.png" srcset="/content/cas/math/people/jcr:content/leftcol/flexmodule.img.418.262.q65.png/1607108331977.png 2x" width="209"/>
Similarly as with links, each image tag has a src
attribute which gives the address where the image file is located.
[62]:
img['src']
[62]:
'/content/cas/math/people/_jcr_content/leftcol/flexmodule.img.209.131.png/1607108331977.png'
We can use requests
to download the image file, and IPython.display
to display it:
[63]:
picture = requests.get('http://www.buffalo.edu' + img['src']).content
with open("ub_logo.png", 'wb') as f:
f.write(picture)
from IPython.display import Image
Image("ub_logo.png", width="50%")
[63]:
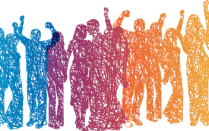
Tables¶
Here is an example of an HTML code defining a simple table:
<table>
<tr>
<th>Item</th>
<th>Quantity</th>
<th>Price</th>
</tr>
<tr>
<td>Tomatoes</td>
<td>5</td>
<td>$3</td>
</tr>
<tr>
<td>Apples</td>
<td>10</td>
<td>$4.50</td>
</tr>
</table>
The whole table is enclosed in the
<table>
tag.Each table row is defined by the
<tr>
tag.Items in the row with table headers are enclosed in the
<th>
tag.Data in all other rows is specified using the
<td>
tag.
Here is the address of a page which shows the table produced by the above code:
https://www.mth548.org/_static/table.html
[39]:
table_url = "https://www.mth548.org/_static/table.html"
IPython.display.IFrame(table_url, width=700, height=85)
[39]:
Let say that we want to use Beautiful Soup to find the quantity and price of apples on the page with the table. First, we get the page content:
[40]:
page = requests.get(table_url).text
table_soup = BeautifulSoup(page)
Next, we find the table tag. Since the page contains only one table, the find()
method can be used for this:
[41]:
table = table_soup.find('table')
table
[41]:
<table style="width: 40%; border-collapse: collapse;">
<tr>
<th>Item</th>
<th>Quantity</th>
<th>Price</th>
</tr>
<tr>
<td>Tomatoes</td>
<td>5</td>
<td>$3</td>
</tr>
<tr>
<td>Apples</td>
<td>10</td>
<td>$4.50</td>
</tr>
</table>
We can find the cell of the table which contains the string “Apples” as follows:
[42]:
for cell in table.find_all('td'):
if "Apples" in cell.get_text():
apples_cell = cell
break
apples_cell
[42]:
<td>Apples</td>
We are interested in the cell appearing immediately after apples_cell
(for quantity), and the one after that (for price). The method find_next()
comes useful here. It searches for the first occurrence of a tag of a specified kind after a given tag:
[43]:
apples_cell.find_next('td') # find the first 'td' tag appearing after apples_cell
[43]:
<td>10</td>
We can chain find_next()
to search for subsequent tags:
[44]:
apples_cell.find_next('td').find_next('td') # find the second 'td' tag appearing after apples_cell
[44]:
<td>$4.50</td>